PHP Client Library for Dialogflow v2 API: Getting started
If you have the choice to go for Python or NodeJS instead of PHP, I recommend using the other two options (between the two, I prefer Python). You will probably find it quite hard to find a lot of discussion online about using PHP with Dialogflow.
This is a very basic tutorial for getting started with the PHP Client Library for Dialogflow API v2. I don’t cover any advanced stuff, but it should help you get started.
1 Create a new folder for your project
In my case, I am just calling it quickstart.

2 Download the client secret JSON file for your v2 Dialogflow agent into the quickstart folder
2.1 Make sure v2 API is enabled
2.2 Click on the service account email address
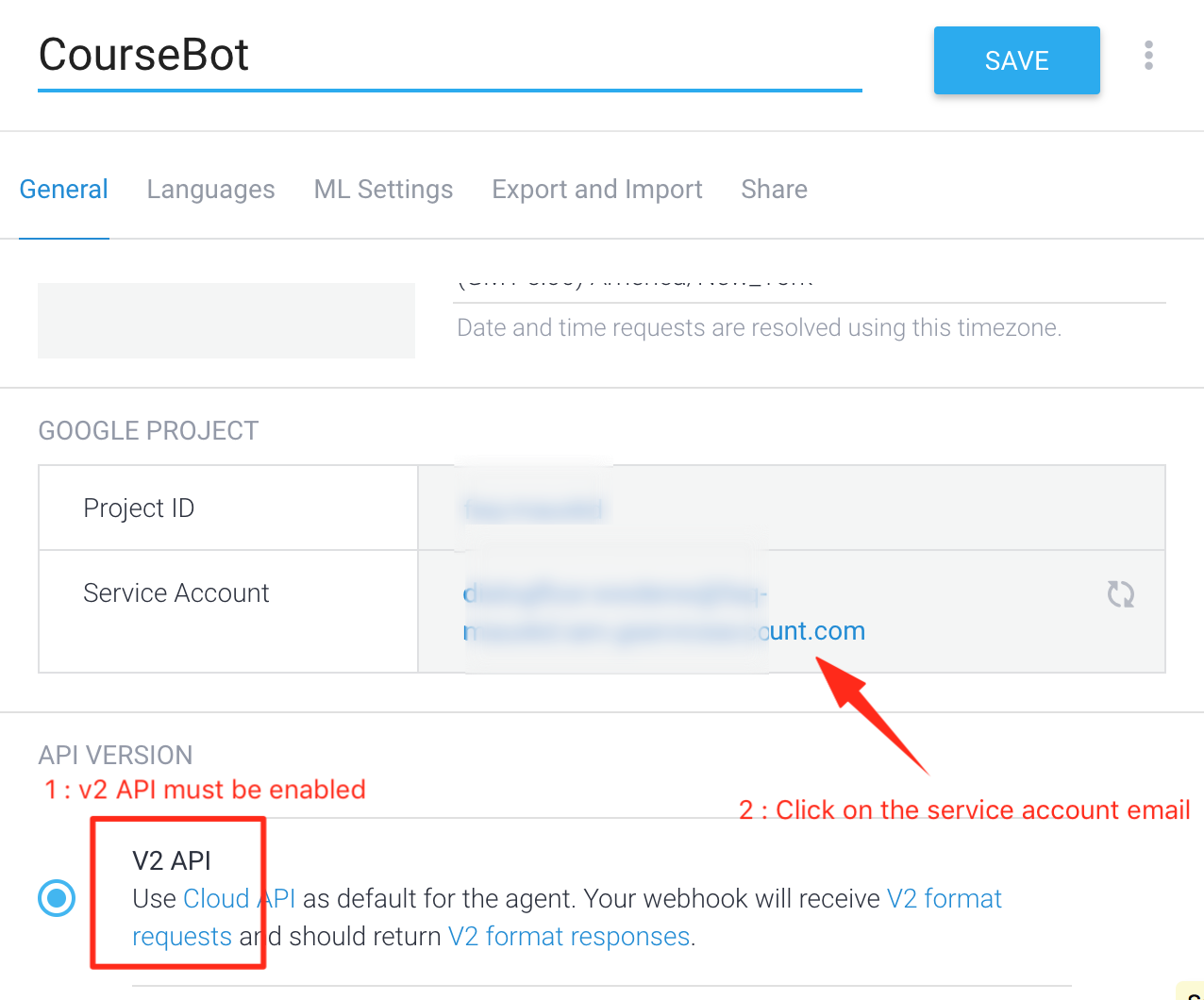
You will be taken to the Google Cloud Console.
2.3 Click on the Create Service Account link at the top of the console menu
2.4 Provide a suitable name for the service account
2.5 Select Project -> Owner in the Role dropdown box
2.6 Make sure you check the Furnish a new private key box. Keep the key type as JSON

2.7 Click on the create link
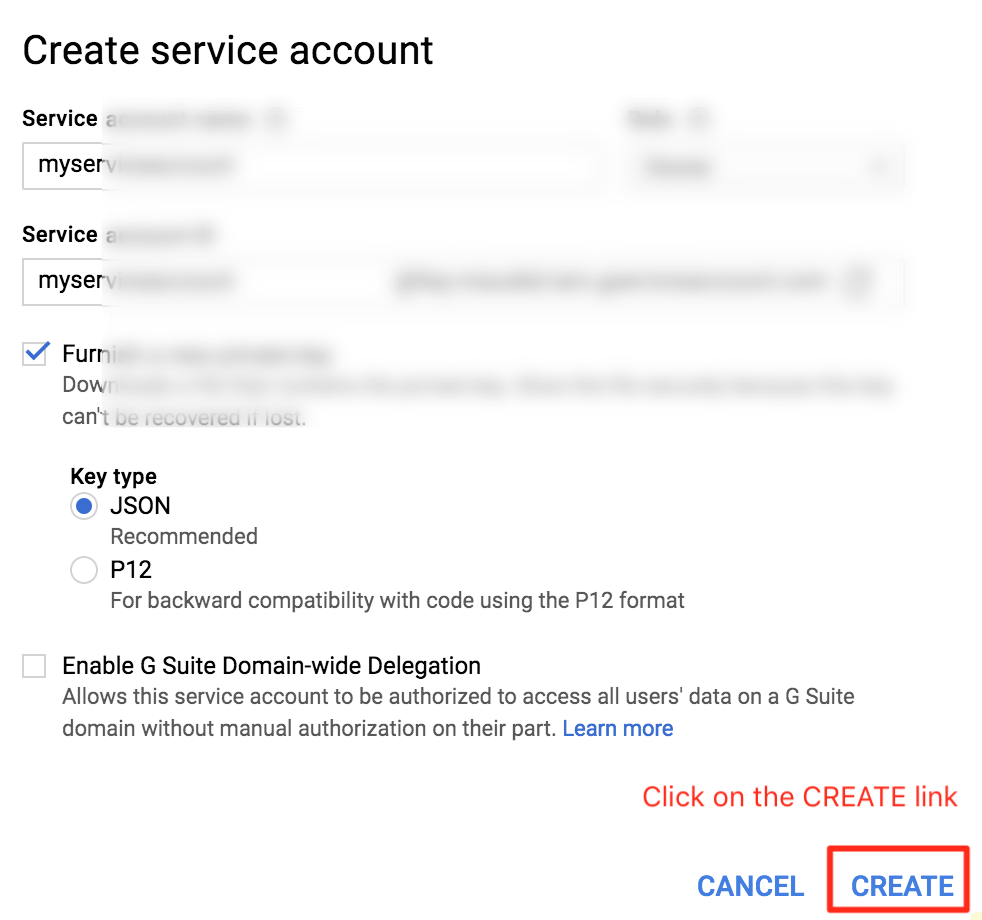
2.8 You will be prompted to save the client secret JSON file. Save the file as client-secret.json to the quickstart folder you just created

New User Interface
Update: After creating this tutorial, I realized that you can do everything here using the Dialogflow API Admin role. The UI has changed a bit now, but essentially you will create a new service account, and choose the role Dialogflow -> API Admin and you will be able to proceed with the rest of the steps in the tutorial. If you are interested in understanding what these roles are, you might want to check out my REST API v2 course.
2.1 Click on Create Service Account

2.2 Select Dialogflow API Admin as the role for the service account

Click on + Create Key button, and select JSON and click on the CREATE button from the slide out. Save the JSON file to the folder.
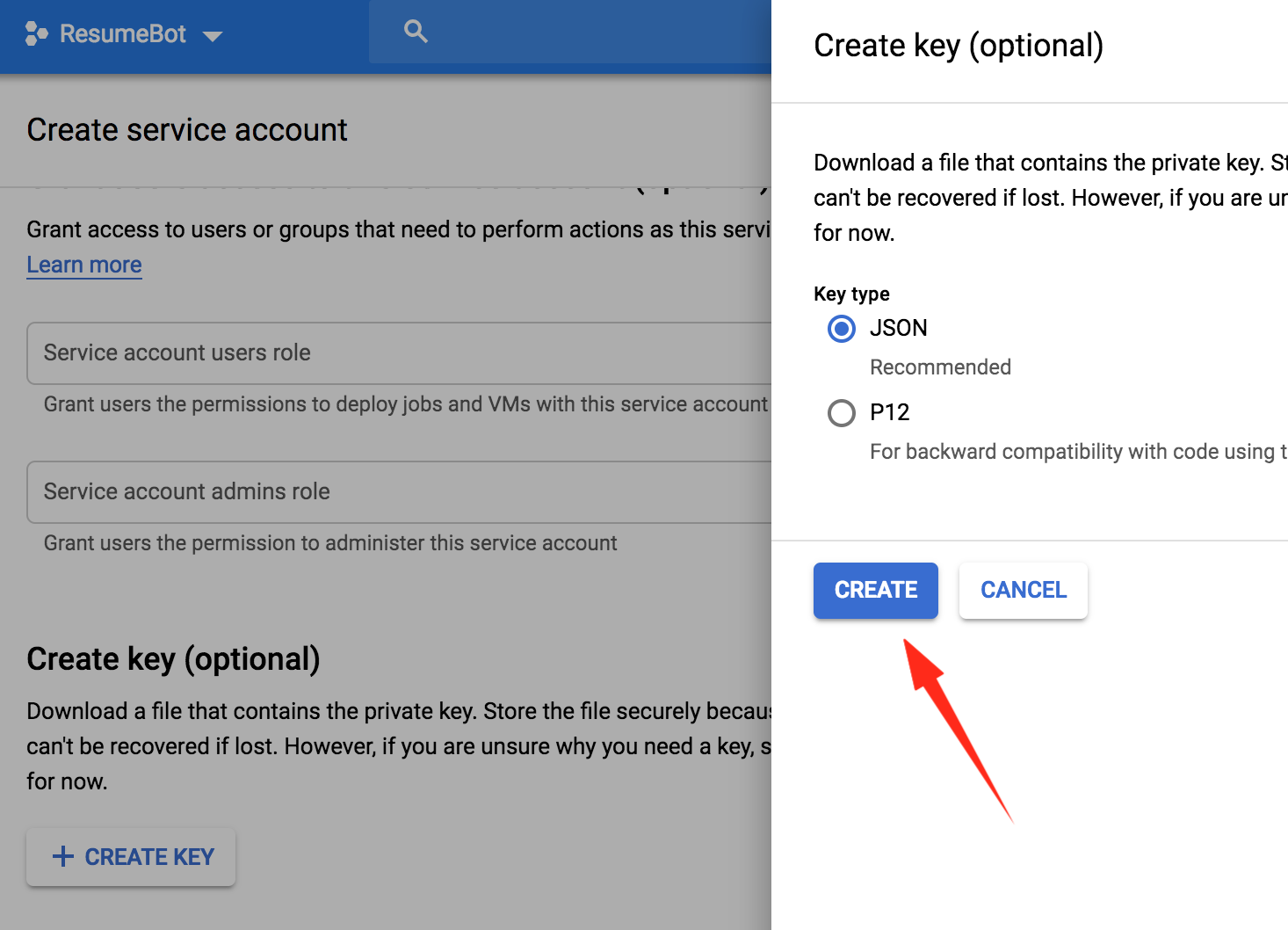
3 Install composer
If you have Homebrew on Mac, you can simply use
brew install composer
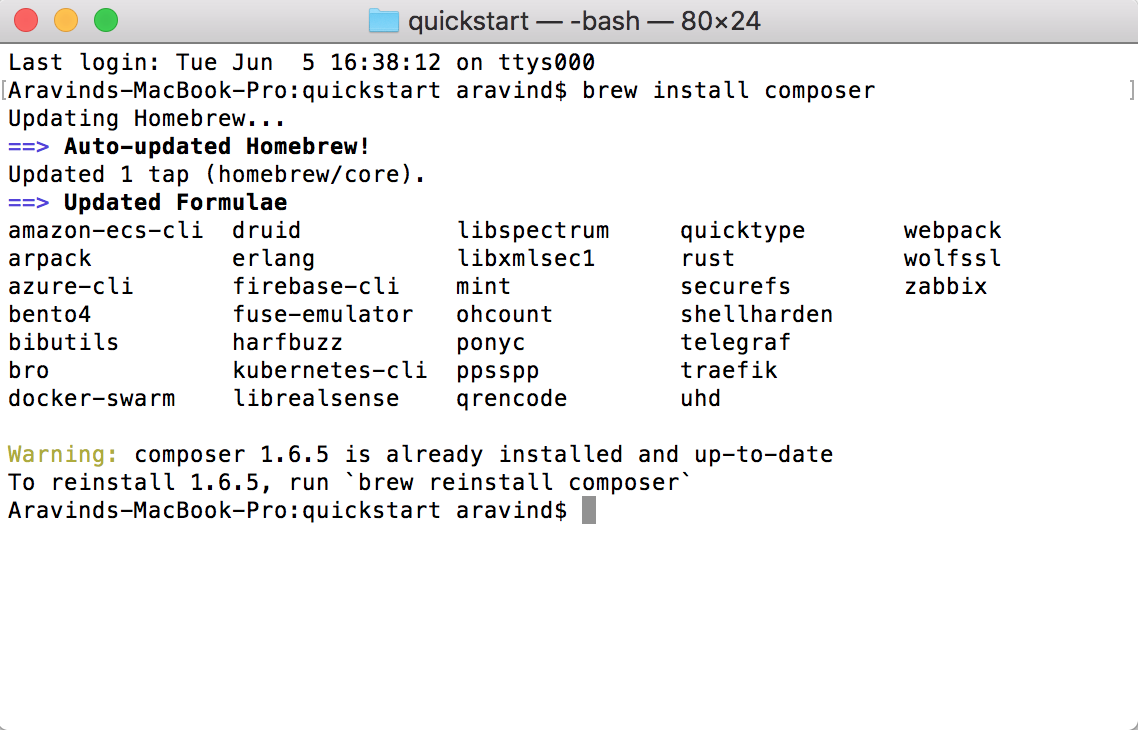
Otherwise you can look online for instructions on how to install composer.
4 Use composer to install Dialogflow client libraries
Before you can use this command, you need to make sure composer was correctly installed. You can use the command
composer require google/cloud-dialogflow
as specified here.
5 PHP Code
5.1 Create an example.php file in the quickstart directory. Your folder structure should look like this at this point. The vendor directory is created when you used composer to install the Dialogflow client libraries.

5.2 Add the required namespaces in example.php
namespace Google\Cloud\Samples\Dialogflow;
use Google\Cloud\Dialogflow\V2\SessionsClient;
use Google\Cloud\Dialogflow\V2\TextInput;
use Google\Cloud\Dialogflow\V2\QueryInput;
5.2 Use Composer’s autoload to add the classes from the vendor directory
require __DIR__.'/vendor/autoload.php';
5.3 Add the following function to example.php
function detect_intent_texts($projectId, $text, $sessionId, $languageCode = 'en-US')
{
// new session
$test = array('credentials' => 'client-secret.json');
$sessionsClient = new SessionsClient($test);
$session = $sessionsClient->sessionName($projectId, $sessionId ?: uniqid());
printf('Session path: %s' . PHP_EOL, $session);
// create text input
$textInput = new TextInput();
$textInput->setText($text);
$textInput->setLanguageCode($languageCode);
// create query input
$queryInput = new QueryInput();
$queryInput->setText($textInput);
// get response and relevant info
$response = $sessionsClient->detectIntent($session, $queryInput);
$queryResult = $response->getQueryResult();
$queryText = $queryResult->getQueryText();
$intent = $queryResult->getIntent();
$displayName = $intent->getDisplayName();
$confidence = $queryResult->getIntentDetectionConfidence();
$fulfilmentText = $queryResult->getFulfillmentText();
// output relevant info
print(str_repeat("=", 20) . PHP_EOL);
printf('Query text: %s' . PHP_EOL, $queryText);
printf('Detected intent: %s (confidence: %f)' . PHP_EOL, $displayName,
$confidence);
print(PHP_EOL);
printf('Fulfilment text: %s' . PHP_EOL, $fulfilmentText);
$sessionsClient->close();
}
5.4 Call the function with the following parameters
detect_intent_texts('your-project-id','hi','123456');
Replace your-project-id with your Dialogflow project ID. The third parameterBoth ES and CX support the concept of parameters. If entitie... More, sessionID, can be any string for this purpose. However, if you are going to be using the client library to manage an entire conversation, your sessionID must be the same across an entire conversation session.
6 Run the code
Now in your Terminal type
php example.php
and you should see the following output. (Make sure you have an actual WelcomeIntent in your Dialogflow agent which has “hi” as one of its training phrases).

Questions/comments?
Leave your feedback in the comments section below.
About this website
I created this website to provide training and tools for non-programmers who are building Dialogflow chatbots.
I have now changed my focus to Vertex AI Search, which I think is a natural evolution from chatbots.
Note
BotFlo was previously called MiningBusinessData. That is why you see that watermark in many of my previous videos.
[17-May-2023 11:58:10 UTC] PHP Fatal error: Uncaught GoogleApiCoreApiException: {
“message”: “com.google.apps.framework.request.NotFoundException: No DesignTimeAgent found for project ‘nies-387004’.”,
“code”: 5,
“status”: “NOT_FOUND”,
“details”: []
}
thrown in /home/nabcbtest/public_html/public/workflow/vendor/google/gax/src/ApiException.php on line 267
Hi there, I have completely stopped using PHP. I suggest asking this on some other forum.
Hello,
I’ve been following this tutorial step-by-step, however my code get stuck when calling “detectIntent” method.
Here’s the code :
$response = $sessionsClient->detectIntent($session, $queryInput);
At this point, my code doesn’t return anything. It also doesn’t return any Exception.
Could you help me, please?
Thanks.
I am moving away from using PHP entirely and concentrating on mostly Python nowadays. So it might be a while before I can get to this. At the moment, I am going to suggest finding other sources to learn this topic.
I find the solution.
It was because I install different Grpc version.
So, I just need to disable Grpc extension on PHP and restart Apache server.
Thanks for your response.
Great! And thanks for the update.
Me tira este error, que puede ser??
Session path: projects/********/agent/sessions/123456
Fatal error: Uncaught GoogleApiCoreApiException: {
“message”: “IAM permission ‘dialogflow.sessions.detectIntent’ on ‘projects/*********/agent’ denied.”,
“code”: 7,
“status”: “PERMISSION_DENIED”,
“details”: []
}
thrown in /Users/***********/Desktop/test/vendor/google/gax/src/ApiException.php on line 139
I am not sure, but the permission issue makes me wonder if you have saved the client-secret JSON file correctly in the same folder as your PHP file and also referenced it with the correct filename in your code.
I have the same issue and my file was correctly named in the code and is present in the same folder than my example.php.
Nevermind I found what is the problem : this is not a name problem. I failed as put myself “owner” as Service account role. Since I was not the owner of my service the acces was denied.
Thanks for the update. I think this will be helpful for others too.
THANK YOU!!! I could not find any clear example on the internet until I got to your page! You are a rockstar!
You are welcome. 🙂
Quick question, do you perhaps know how to set context with this? I have tried everything. I included the ” use GoogleCloudDialogflowV2Context;” in the code and then added the following, but it still fails. Please tell me you know how to do this?
// $context is a normal php array containing the values
$contextInput = new Context();
$contextInput->setLifespanCount($context[‘lifespan’]);
$contextInput->setName($context[‘name’]);
$contextInput->setParameters($context[‘parameters’]);
Nevermind, my friend. I figured it out. In case anyone else needs this. Here is what I did.
I added these as well:
use GoogleProtobufValue;
use GoogleProtobufStruct;
Then I created my context like this
$contextInput = new Context();
$contextInput->setLifespanCount($context[‘lifespan’]);
$contextInput->setName($context[‘name’]);
$contextValue = new Value();
$context[‘parameters’][‘number_param_key’] = $contextValue->setNumberValue($context[“parameters”][“number_param”]); // In my case I was passing a number
$contextStruct = new Struct();
$contextStruct->setFields($context[‘parameters’]);
$contextInput->setParameters($contextStruct);
This seems to have worked and my agent is chatting again with the context set !
Thanks anyway!
Hi Christopher,
What are the exact contents of $context? Specifically when you call ‘$contextStruct->setFields($context[‘parameters’]);’ what is passed as $context[‘parameters’]?
Hey it’s me again and amidst this corona scare nobody from support helped out. And their docs are cryptic as shit. So I figured it out:
// create query input
$queryInput = new QueryInput();
$queryInput->setText($textInput);
//$contextPath = $session . ‘/contexts/’ . ‘masters’;
$contextPath = $session . ‘/contexts/’ . $contextText;
$context = new Context();
$context->setName($contextPath);
$context->setLifespanCount(1);
$queryParams = new QueryParameters([‘contexts’ => [$context]]);
// get response and relevant info
$response = $sessionsClient->detectIntent($session, $queryInput, [‘queryParams’ => $queryParams]);
Hello,
How i can get a JSON response? 🙂
Like this:
{
“responseId”: “ecffd044-d4b4-4613-978e-23b69dcc3110-f6406966”,
“queryResult”: {
“fulfillmentMessages”: [
{
“platform”: “FACEBOOK”,
“text”: {
“text”: [
“Hallo!nDit is een test werkt dit o”
]
},
“message”: “text”
},
{
“platform”: “FACEBOOK”,
“quickReplies”: {
“quickReplies”: [
“Nee”
],
“title”: “Ja”
},
“message”: “quickReplies”
},
{
“platform”: “PLATFORM_UNSPECIFIED”,
“text”: {
“text”: [
“Hallo!nDit is een test werkt dit o”
]
},
“message”: “text”
}
],
“outputContexts”: [],
“queryText”: “Hello je meoder”,
“speechRecognitionConfidence”: 0,
“action”: “input.welcome”,
“parameters”: {
“fields”: {}
},
“allRequiredParamsPresent”: true,
“fulfillmentText”: “Hallo!nDit is een test werkt dit o”,
“webhookSource”: “”,
“webhookPayload”: null,
“intent”: {
“inputContextNames”: [],
“events”: [],
“trainingPhrases”: [],
“outputContexts”: [],
“parameters”: [],
“messages”: [],
“defaultResponsePlatforms”: [],
“followupIntentInfo”: [],
“name”: “projects/livebot-coftjp/agent/intents/70e47523-f9e3-402f-a1fc-20c496d5d846”,
“displayName”: “Default Welcome Intent”,
“priority”: 0,
“isFallback”: false,
“webhookState”: “WEBHOOK_STATE_UNSPECIFIED”,
“action”: “”,
“resetContexts”: false,
“rootFollowupIntentName”: “”,
“parentFollowupIntentName”: “”,
“mlDisabled”: false
},
“intentDetectionConfidence”: 0.3702174425125122,
“diagnosticInfo”: null,
“languageCode”: “nl”
},
“webhookStatus”: null
}
As Nike says: Just do it. 🙂
I am not sure what you are asking here.
Thanks you!! I was searching about it and google not give a clear information!
Hi, Can I use this code to run on a server and call this example.php from chrome? I have checked this way but not working. I have also checked using putty. It also giving no output as well as no error. Any suggestions, please?
Just to clarify: you mean it works as described on your local machine, but fails when you upload to the server?
Yes, I am getting it on local machine. But now I think the server I have used is without SSL. Am I right that it will only work in “https” domain? But not any errors are listing!
To be honest, I am not entirely sure and it is quite hard to debug this. There are a couple of options: you can schedule a paid consultation if it is urgent. If not, give me a week or so, and I will create a tutorial which will use Heroku (and will be more easy to deploy to web).
Holes, espero q estes bien. Estoy explorando DialogFlow como solución, estoy consumiendo la API Rest con PHP, DialogFlow versión free usando este tutorial muy útil.
Tengo un síntoma, consiste que solo tengo respuesta con la intensión ‘Default Welcome Intent’, en una intensión diferente me arroja un error. Ajunto pantallazo:
En los 2 primeras peticiones va con frase de entrenamiento de intensión ‘Default Welcome Intent’, el cual hay respuesta satisfactoria.
En la 3 petición, va con una frase de entrenamiento de una intensión nueva, pero arroja un error.
Nota: Las 3 peticiones contiene el mismo sessionId. Al final del email esta en detalle el error.
Gracias,
[root@centos-s-1vcpu-1gb-sgp1-01 dialogflow]# php Calldiaglogflow.php
Session path: projects/agente-virtual-acfff/agent/sessions/12345678
Hola! Soy asistente virtual IngeTecno, Donde vives ?[root@centos-s-1vcpu-1gb-sgp1-01 dialogflow]#
[root@centos-s-1vcpu-1gb-sgp1-01 dialogflow]#
[root@centos-s-1vcpu-1gb-sgp1-01 dialogflow]# php Calldiaglogflow.php
Session path: projects/agente-virtual-acfff/agent/sessions/12345678
Hola! Soy asistente virtual IngeTecno, Donde vives ?[root@centos-s-1vcpu-1gb-sgp1-01 dialogflow]#
[root@centos-s-1vcpu-1gb-sgp1-01 dialogflow]#
[root@centos-s-1vcpu-1gb-sgp1-01 dialogflow]# php Calldiaglogflow.php
Session path: projects/agente-virtual-acfff/agent/sessions/12345678
PHP Fatal error: Uncaught Error: Call to undefined function GoogleProtobufInternalbccomp() in /var/lib/asterisk/agi-bin/dialogflow/vendor/google/protobuf/src/Google/Protobuf/Internal/Message.php:900
Stack trace:
#0 /var/lib/asterisk/agi-bin/dialogflow/vendor/google/protobuf/src/Google/Protobuf/Internal/Message.php(1218): GoogleProtobufInternalMessage->convertJsonValueToProtoValue(4, Object(GoogleProtobufInternalFieldDescriptor))
#1 /var/lib/asterisk/agi-bin/dialogflow/vendor/google/protobuf/src/Google/Protobuf/Internal/Message.php(1167): GoogleProtobufInternalMessage->mergeFromArrayJsonImpl(Array)
#2 /var/lib/asterisk/agi-bin/dialogflow/vendor/google/protobuf/src/Google/Protobuf/Internal/Message.php(815): GoogleProtobufInternalMessage->mergeFromJsonArray(Array)
#3 /var/lib/asterisk/agi-bin/dialogflow/vendor/google/protobuf/src/Google/Protobuf/Internal/Message.php(1218): GoogleProtobufInternalMessage->convertJsonValueToProtoValue(Array, Object(GoogleProtobufInternalFieldDescriptor))
#4 /var/lib/asterisk/ag in /var/lib/asterisk/agi-bin/dialogflow/vendor/google/protobuf/src/Google/Protobuf/Internal/Message.php on line 900
I used Google Translate and didn’t really understand your full question. You need to ask in English so I can understand the full meaning.
I’m using local and I have the same problem of having no answer. No error appears, just returns nothing in the terminal.
OK, guess I have to get going on that Heroku based tutorial sooner. 🙂
My code worked. The new problem is that I have to run it with PHP in version 5.4. I could not see any examples. Are there any possibilities to run Dialogflow with PHP in version 5.4.16?
The code works for PHP 7. I don’t know the answer to your question unfortunately.
Thanks.
Is there any course that will fully explain about v2 as well as teaches how to create chatbot using v2 php sdk from scratch
Well, looks like you already found and purchased the Dialogflow REST API v2 course. Although note that the course teaches you the fundamentals and is programming-language agnostic.
Been looking for straight forward way to use dialogflow API from an external page. I’m glad that I’ve found your article, thanks a lot!
It’s Not Work
Still works for me, I just checked.
it’s working . sorry my misteke
Do you know how one would extract the audio using the php library? (ie. using the beta text to audio function in dialogflow?)
Sorry, I haven’t worked on that till now.
its me again haha.
is there a way to get multiple responses with PHP SDK?
everytime, i just get the first response
I am not sure. I do know that sometimes it helps to make the call to the API directly from PHP code rather than using the SDK, and I explain how to do that in my REST API course. That might help in your case.
Hi its me again,
i got the following error
Uncaught PHP Exception GoogleApiCoreValidationException: “Cannot bind segment ‘{project=*}’ to value ”” at
Its hard to say, but it looks like you have some error in the URL endpoint you are using. Maybe someone else will pitch in if they have run into the same issue.
i searchedit on google and cannot find any response or similiar
by endpoint, what do you mean?
this is the entire error.
request.CRITICAL: Uncaught PHP Exception GoogleApiCoreValidationException: “Cannot bind segment ‘{project=*}’ to value ”” at /opt/sdp/gchat/www/chatgenerico/vendor/google/gax/src/ApiCore/ResourceTemplate/Segment.php line 121 {“exception”:”[object] (Google\ApiCore\ValidationException(code: 0): Cannot bind segment ‘{project=*}’ to value ” at /opt/sdp/gchat/www/chatgenerico/vendor/google/gax/src/ApiCore/ResourceTemplate/Segment.php:121)”} []
I meant that something might be wrong with the structure of the URL you are calling to access the API. I haven’t seen this error, so I am not sure what is causing it.
how i use this to chat dynamically
You need to write your own custom code to do this, and use the ideas described here for the detectIntent functionality.
i run this in terminal its good.. but how can i run this like real chat on browser
Take a look here for some ideas. You need to write your own custom code for this.
hi , is it there a way to handle promtp responses(when a parameters is missing) with this php sdk?
ty
I wrote a response here.
How to add knowledge bases params to queryParameters class??
I don’t even see any reference to knowledgebases in the v2 client library at the moment. You probably have to use direct REST API calls in your PHP code if you want to access these v2 API methods as of this writing.
Been trying to get this working. Keep running into permission issues. I enabled the dialogflow v2 API, created the service account, added the owner role to it, downloaded the JSON key…and I keep getting this as a response:
PHP Fatal error: Uncaught GoogleApiCoreApiException: {
“message”: “IAM permission ‘dialogflow.sessions.detectIntent’ on ‘projects/dialogflow-XXXXXX/agent’ denied.”,
“code”: 7,
“status”: “PERMISSION_DENIED”,
“details”: []
}
I will look into this tomorrow
I just tried it now, and it still works as described in this tutorial. My suggestion would be to start the project in a completely new directory, reinstall the libraries using composer and try again (in case the libraries got messed up somehow). One more thing you might want to check is to see if the Dialogflow API is enabled for your project (login to Google Cloud Console -> APIs and services from left menu -> Dashboard).
It ended up being something weird on Google’s end. I deleted the Service Account, started fresh and everything worked. Thanks!
I have created a test chatbot and enabled V2 API and then created the service account by following above steps.
After that I have installed V2 client library using composer and added the client-secret.json file in folder directory.
While trying to use : composer require __DIR__.’/vendor/autoload.php’;
getting below error:
[InvalidArgumentException]
Could not find a matching version of package __DIR__.’/vendor/autoload.php’
. Check the package spelling, your version constraint and that the package
is available in a stability which matches your minimum-stability (stable).
Please help
To clarify, the line
require __DIR__.’/vendor/autoload.php’;
goes inside your PHP code, right after the namespace section. Other than that, I am not sure what is causing the error, you might have to ask on some other forum.
Can you help please, I am trying to get the data from:
$queryResult->getFulfillmentMessages();
but I can’t.
I tried:
json_decode($queryResult->getFulfillmentMessages()->serializeToJsonString(), true);
but show me a error, serializeToJsonString doesn’t exist.
I really will apreciate it.
Can you first assign a var e.g. $fm = $queryResult->getFulfillmentMessages() and see what value gets assigned to it? Is it empty?
Hey Walter,
This solution worked for me. The response that is received from the queryResult is a protobuf repeated field. The payload that is required to be extracted can be accessed by calling the first element of the repeated field and serializing it to JSON string then decoding it.
json_decode($queryResult->getFulfillmentMessages()[0]->serializeToJsonString(), true);
This will give the payload in array format using which you can perform your operations on it.
This is very helpful. Thanks for the comment.
How to use PHP V2 Client Libraries to create a chatbot. Kindly guide.
Please take a look here.